Summary: in this tutorial, you will learn how to use the SQL Server OR
operator to combine two Boolean expressions.
Introduction to SQL Server OR operator
The SQL Server OR
is a logical operator that allows you to combine two Boolean expressions. It returns TRUE
when either of the conditions evaluates to TRUE
.
The following shows the syntax of the OR
operator:
boolean_expression OR boolean_expression
Code language: SQL (Structured Query Language) (sql)
In this syntax, the boolean_expression
is any valid Boolean expression that evaluates to true, false, and unknown.
The following table shows the results of the OR
operator when you combine TRUE
, FALSE
, and UNKNOWN
:
TRUE | FALSE | UNKNOWN | |
---|---|---|---|
TRUE | TRUE | TRUE | TRUE |
FALSE | TRUE | FALSE | UNKNOWN |
UNKNOWN | TRUE | UNKNOWN | UNKNOWN |
When you use multiple logical operators in an expression, SQL Server always evaluates the OR
operators after AND
operators. But you can use the parentheses ()
to change the order of the evaluation.
SQL Server OR operator examples
We’ll use the following production.roducts
table from the sample database.
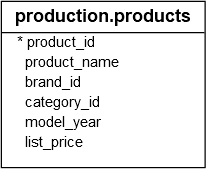
1) Basic SQL Server OR operator example
The following example uses the OR operator to find the products whose list price is less than 200 or greater than 6,000:
SELECT
product_name,
list_price
FROM
production.products
WHERE
list_price < 200
OR list_price > 6000
ORDER BY
list_price;
Code language: SQL (Structured Query Language) (sql)
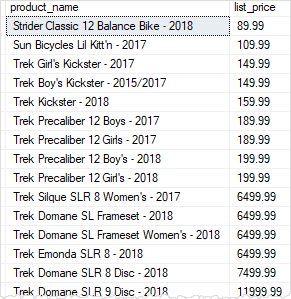
2) Using multiple OR operators
The following statement uses multiple OR operators to find the products whose brand id is 1, 2, or 4:
SELECT
product_name,
brand_id
FROM
production.products
WHERE
brand_id = 1
OR brand_id = 2
OR brand_id = 4
ORDER BY
brand_id DESC;
Code language: SQL (Structured Query Language) (sql)
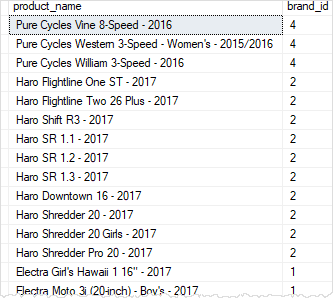
You can replace multiple OR
operators by the IN
operator as shown in the following query:
SELECT
product_name,
brand_id
FROM
production.products
WHERE
brand_id IN (1, 2, 3)
ORDER BY
brand_id DESC;
Code language: SQL (Structured Query Language) (sql)
3) Combining the OR operator with the AND operator
The following example shows how to combine the OR
operator with the AND
operator within the same expression:
SELECT
product_name,
brand_id,
list_price
FROM
production.products
WHERE
brand_id = 1
OR brand_id = 2
AND list_price > 500
ORDER BY
brand_id DESC,
list_price;
Code language: SQL (Structured Query Language) (sql)
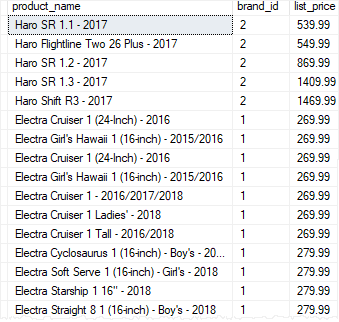
In this example, we used both OR
and AND
operators. As always, SQL Server evaluated the AND
operator first. Therefore, the query returned the products whose brand id is 2 and the list price is greater than 500 or those whose brand id is 1.
To find the products whose brand id is 1 or 2 and list price is greater than 500, you use the parentheses as shown in the following query:
SELECT
product_name,
brand_id,
list_price
FROM
production.products
WHERE
(brand_id = 1 OR brand_id = 2)
AND list_price > 500
ORDER BY
brand_id;
Code language: SQL (Structured Query Language) (sql)
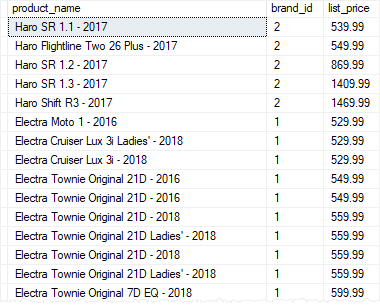
Summary
- Use the SQL Server
OR
operator to combine two Boolean expressions. - The
OR
operator returnsTRUE
if one of the expressions isTRUE
. - By default, SQL Server evaluates the
OR
operators after theAND
operators within the same expression. But you can use parentheses()
to change the order of evaluation.